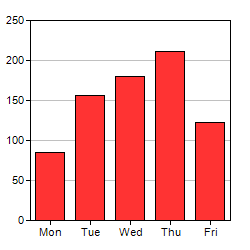
This section introduces using ChartDirector in ASP.NET Web Forms. For ASP.NET MVC / Razor Pages, please refer to The First ASP.NET MVC / Razor Pages Project. For Windows Forms, please refer to The First Windows Forms Project. For WPF, please refer to The First WPF Project.
To get a feeling of using ChartDirector, and to verify the ChartDirector development environment is set up properly, we will begin by building a very simple bar chart.
The following code module comes from the "NetWebCharts" sample programs included in the ChartDirector distribution. If you have not yet tried the sample programs, it is highly recommended you try them now. Please refer to the Installation section for details. They are very useful for exploring and testing the features of ChartDirector.
[ASP.NET Web Forms - C# version] NetWebCharts\CSharpASP\simplebar.aspx
(Click here on how to convert this code to code-behind style.)
<%@ Page Language="C#" Debug="true" %>
<%@ Import Namespace="ChartDirector" %>
<%@ Register TagPrefix="chart" Namespace="ChartDirector" Assembly="netchartdir" %>
<!DOCTYPE html>
<script runat="server">
//
// Page Load event handler
//
protected void Page_Load(object sender, EventArgs e)
{
// The data for the bar chart
double[] data = {85, 156, 179.5, 211, 123};
// The labels for the bar chart
string[] labels = {"Mon", "Tue", "Wed", "Thu", "Fri"};
// Create a XYChart object of size 250 x 250 pixels
XYChart c = new XYChart(250, 250);
// Set the plotarea at (30, 20) and of size 200 x 200 pixels
c.setPlotArea(30, 20, 200, 200);
// Add a bar chart layer using the given data
c.addBarLayer(data);
// Set the labels on the x axis.
c.xAxis().setLabels(labels);
// Output the chart
WebChartViewer1.Image = c.makeWebImage(Chart.SVG);
// Include tool tip for the chart
WebChartViewer1.ImageMap = c.getHTMLImageMap("", "", "title='{xLabel}: {value} GBytes'");
}
</script>
<html>
<head>
<script type="text/javascript" src="cdjcv.js"></script>
</head>
<body>
<chart:WebChartViewer id="WebChartViewer1" runat="server" />
</body>
</html>
[ASP.NET Web Forms - VB Version] NetWebCharts\VBNetASP\simplebar.aspx
(Click here on how to convert this code to code-behind style.)
<%@ Page Language="VB" Debug="true" %>
<%@ Import Namespace="ChartDirector" %>
<%@ Register TagPrefix="chart" Namespace="ChartDirector" Assembly="netchartdir" %>
<!DOCTYPE html>
<script runat="server">
'
' Page Load event handler
'
Protected Sub Page_Load(ByVal sender As System.Object, ByVal e As System.EventArgs)
' The data for the bar chart
Dim data() As Double = {85, 156, 179.5, 211, 123}
' The labels for the bar chart
Dim labels() As String = {"Mon", "Tue", "Wed", "Thu", "Fri"}
' Create a XYChart object of size 250 x 250 pixels
Dim c As XYChart = New XYChart(250, 250)
' Set the plotarea at (30, 20) and of size 200 x 200 pixels
c.setPlotArea(30, 20, 200, 200)
' Add a bar chart layer using the given data
c.addBarLayer(data)
' Set the labels on the x axis.
c.xAxis().setLabels(labels)
' Output the chart
WebChartViewer1.Image = c.makeWebImage(Chart.SVG)
' Include tool tip for the chart
WebChartViewer1.ImageMap = c.getHTMLImageMap("", "", "title='{xLabel}: {value} GBytes'")
End Sub
</script>
<html>
<head>
<script type="text/javascript" src="cdjcv.js"></script>
</head>
<body>
<chart:WebChartViewer id="WebChartViewer1" runat="server" />
</body>
</html>
The code is explained below:
- To use ChartDirector conveniently, the program imports the ChartDirector namespace using the following code.
<%@ Import Namespace=
"ChartDirector" %>
If you are using Visual Studio, and the source code is in a code-behind module, you should import the ChartDirector namespace in the code-behind module using:
- In this project, we use the WebChartViewer control for viewing the chart. The WebChartViewer control is a server side Web Forms control. To use it, we need to register a tag prefix for the control using the following code.
<%@Register TagPrefix=
"chart" Namespace= "ChartDirector" Assembly= "netchartdir"%>
After registration, we can include the WebChartViewer control in the HTML portion of the code as follows:
<chart:WebChartViewer id=
"WebChartViewer1" runat= "server" />
If you are using Visual Studio, you can simply drag and drop the WebChartViewer from the Toolbox to your Web Form. Visual Studio will automatically create the "<%@Register%>" and "<chart:WebChartViewer>" tags.
- The first step in creating any chart in ChartDirector is to create the appropriate chart object. In this example, an XYChart object of size 250 x 250 pixels is created. In ChartDirector, XYChart represents any chart that has x-axis and y-axis, such as the bar chart we are drawing.
[VB]
Dim c As New XYChart(
250, 250) [C#]
XYChart c = new XYChart(
250, 250);
- The second step in creating a bar chart is to specify where should we draw the bar chart. This is by specifying the rectangle that contains the bar chart. The rectangle is specified by using the (x, y) coordinates of its top-left corner, together with its width and height.
[VB]
c.setPlotArea(
30, 20, 200, 200) [C#]
c.setPlotArea(
30, 20, 200, 200);
For this simple bar chart, we will use the majority of the chart area to draw the bar chart. We will leave some margin to allow for the text labels on the axis. In the above code, the top-left corner is set to (30, 30), and both the width and height is set to 200 pixels. Since the entire chart is 250 x 250 in size, there will be 20 to 30 pixels margin for the text labels.
Note that ChartDirector uses a pixel coordinate system that is customary for computer screen. The x pixel coordinate is increasing from left to right. The y pixel coordinate is increasing from top to bottom. The origin (0, 0) is at the top-left corner.
For more complex charts which may contain titles, legend box and other things, we can use this method (and other methods) to design the exact layout of the entire chart.
- After the plot area is specified, we can add a bar chart layer to the XYChart.
[VB]
c.addBarLayer(
data) [C#]
c.addBarLayer(
data);
The above code adds a bar layer to the XYChart. In ChartDirector, any chart type that has x-axis and y-axis is represented as a layer in the XYChart. An XYChart can contain multiple layers. This allows "combination charts" to be created easily by combining different layers on the same chart (eg., a chart containing a line layer on top of a bar layer) .
In the above line of code, the argument is an array of numbers representing the values of the data points.
- By default, ChartDirector will use "auto-scaling" for the y-axis. On the other hand, for the x-axis, we need to specify the labels as they are text strings.
[VB]
c.xAxis(
).setLabels( labels) [C#]
c.xAxis(
).setLabels( labels);
The above code sets the labels on the x-axis. The first method XYChart.xAxis retrieves the Axis object that represents the x-axis. The second method Axis.setLabels binds the text labels to the x-axis. The argument to the setLabels method is an array of text strings.
- Up to this point, the chart is completed. In this project, we will output the chart to the browser as a SVG image by using the WebChartViewer control.
[VB]
WebChartViewer1.Image = c.makeWebImage(
Chart.SVG) [C#]
WebChartViewer1.Image = c.makeWebImage(
Chart.SVG);
The WebChartViewer control is a server side Web Forms control that will translate the chart image into an <IMG> tag on the browser side. It will then send the chart image to the browser to fulfill the <IMG> tag "on the fly" (without creating any temporary file).
Besides sending the chart image to the browser using WebChartViewer, ChartDirector also supports other chart output methods (eg. creating the chart as an image file). These methods and their applications will be described in ChartDirector Output Methods.
- After displaying the chart, an image map can be created for the chart to support mouse interactions. In this example, the image map is configured to display tool tips of the format
"title='{xLabel}: {value} GBytes'"
when the mouse cursor is on top of the bars.
[VB]
WebChartViewer1.ImageMap = c.getHTMLImageMap(
"", "", "title= '{xLabel}: {value} GBytes'") [C#]
WebChartViewer1.ImageMap = c.getHTMLImageMap(
"", "", "title= '{xLabel}: {value} GBytes'");
- The HTML part of the sample code is just a basic web page. Note that we included the ChartDirector Javascript Library in the web page. This library provides support for other advanced ChartDirector features, such as responsive design, CDML tooltips, real-time update, programmable track cursor and zooming and scrolling. Although it is not needed for this simple example, we suggest to include "cdjcv.js" in all web pages using ChartDirector.
Note: The trial version of ChartDirector will include small yellow banners at the bottom of the charts it produces. These banners will disappear in the licensed version of ChartDirector.