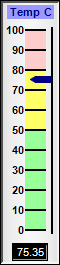
This example demonstrates a simple vertical linear meter.
A basic linear meter can be created using the following steps:
- Create a LinearMeter object using LinearMeter.LinearMeter. In this example, the background color is set to silver using Chart.silverColor and the meter border is set to have a depressed effect.
- Specify the size of the meter scale region using LinearMeter.setMeter and the orientation of the scale labels. In this example, the scale labels are set at the left of the meter scale region. This automatically sets the meter as vertical.
- Set the numeric scale of the meter using BaseMeter.setScale
- Add a pointer to the meter using BaseMeter.addPointer
- Define zones on the meter using LinearMeter.addZone. In this example, 3 zones (red, yellow and green) are defined.
- Add arbitrary text boxes to the meter using BaseChart.addText. In this example, a text box is added to specify the meter as showing temperature, and another text box is added to display the data value as text.