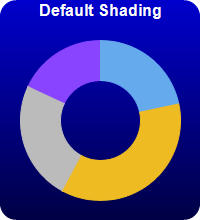

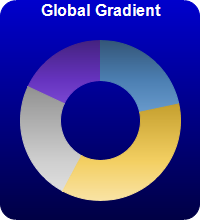


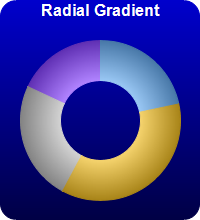
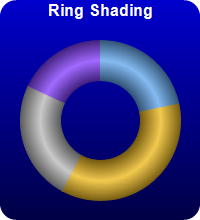
This example demonstrates various sector shading effects applicable to 2D donut charts.
ChartDirector 5.1 (.NET Edition)
2D Donut Shading
Source Code Listing
<%@ Page Language="VB" Debug="true" %> <%@ Import Namespace="ChartDirector" %> <%@ Register TagPrefix="chart" Namespace="ChartDirector" Assembly="netchartdir" %> <script runat="server"> ' ' Create chart ' Protected Sub createChart(viewer As WebChartViewer, img As String) ' The data for the pie chart Dim data() As Double = {18, 30, 20, 15} ' The labels for the pie chart Dim labels() As String = {"Labor", "Licenses", "Facilities", "Production"} ' The colors to use for the sectors Dim colors() As Integer = {&H66aaee, &Heebb22, &Hbbbbbb, &H8844ff} ' Create a PieChart object of size 200 x 220 pixels. Use a vertical gradient ' color from blue (0000cc) to deep blue (000044) as background. Use rounded ' corners of 16 pixels radius. Dim c As PieChart = New PieChart(200, 220) c.setBackground(c.linearGradientColor(0, 0, 0, c.getHeight(), &H0000cc, _ &H000044)) c.setRoundedFrame(&Hffffff, 16) ' Set donut center at (100, 120), and outer/inner radii as 80/40 pixels c.setDonutSize(100, 120, 80, 40) ' Set the pie data c.setData(data, labels) ' Set the sector colors c.setColors2(Chart.DataColor, colors) ' Demonstrates various shading modes If img = "0" Then c.addTitle("Default Shading", "bold", 12, &Hffffff) ElseIf img = "1" Then c.addTitle("Local Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.LocalGradientShading) ElseIf img = "2" Then c.addTitle("Global Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.GlobalGradientShading) ElseIf img = "3" Then c.addTitle("Concave Shading", "bold", 12, &Hffffff) c.setSectorStyle(Chart.ConcaveShading) ElseIf img = "4" Then c.addTitle("Rounded Edge", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RoundedEdgeShading) ElseIf img = "5" Then c.addTitle("Radial Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RadialShading) ElseIf img = "6" Then c.addTitle("Ring Shading", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RingShading) End If ' Disable the sector labels by setting the color to Transparent c.setLabelStyle("", 8, Chart.Transparent) ' Output the chart viewer.Image = c.makeWebImage(Chart.PNG) ' Include tool tip for the chart viewer.ImageMap = c.getHTMLImageMap("", "", _ "title='{label}: US${value}K ({percent}%)'") End Sub ' ' Page Load event handler ' Protected Sub Page_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) createChart(WebChartViewer0, "0") createChart(WebChartViewer1, "1") createChart(WebChartViewer2, "2") createChart(WebChartViewer3, "3") createChart(WebChartViewer4, "4") createChart(WebChartViewer5, "5") createChart(WebChartViewer6, "6") End Sub </script> <html> <body> <chart:WebChartViewer id="WebChartViewer0" runat="server" /> <chart:WebChartViewer id="WebChartViewer1" runat="server" /> <chart:WebChartViewer id="WebChartViewer2" runat="server" /> <chart:WebChartViewer id="WebChartViewer3" runat="server" /> <chart:WebChartViewer id="WebChartViewer4" runat="server" /> <chart:WebChartViewer id="WebChartViewer5" runat="server" /> <chart:WebChartViewer id="WebChartViewer6" runat="server" /> </body> </html> |
<%@ Page Language="C#" Debug="true" %> <%@ Import Namespace="ChartDirector" %> <%@ Register TagPrefix="chart" Namespace="ChartDirector" Assembly="netchartdir" %> <script runat="server"> // // Create chart // protected void createChart(WebChartViewer viewer, string img) { // The data for the pie chart double[] data = {18, 30, 20, 15}; // The labels for the pie chart string[] labels = {"Labor", "Licenses", "Facilities", "Production"}; // The colors to use for the sectors int[] colors = {0x66aaee, 0xeebb22, 0xbbbbbb, 0x8844ff}; // Create a PieChart object of size 200 x 220 pixels. Use a vertical gradient // color from blue (0000cc) to deep blue (000044) as background. Use rounded // corners of 16 pixels radius. PieChart c = new PieChart(200, 220); c.setBackground(c.linearGradientColor(0, 0, 0, c.getHeight(), 0x0000cc, 0x000044) ); c.setRoundedFrame(0xffffff, 16); // Set donut center at (100, 120), and outer/inner radii as 80/40 pixels c.setDonutSize(100, 120, 80, 40); // Set the pie data c.setData(data, labels); // Set the sector colors c.setColors2(Chart.DataColor, colors); // Demonstrates various shading modes if (img == "0") { c.addTitle("Default Shading", "bold", 12, 0xffffff); } else if (img == "1") { c.addTitle("Local Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.LocalGradientShading); } else if (img == "2") { c.addTitle("Global Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.GlobalGradientShading); } else if (img == "3") { c.addTitle("Concave Shading", "bold", 12, 0xffffff); c.setSectorStyle(Chart.ConcaveShading); } else if (img == "4") { c.addTitle("Rounded Edge", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RoundedEdgeShading); } else if (img == "5") { c.addTitle("Radial Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RadialShading); } else if (img == "6") { c.addTitle("Ring Shading", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RingShading); } // Disable the sector labels by setting the color to Transparent c.setLabelStyle("", 8, Chart.Transparent); // Output the chart viewer.Image = c.makeWebImage(Chart.PNG); // Include tool tip for the chart viewer.ImageMap = c.getHTMLImageMap("", "", "title='{label}: US${value}K ({percent}%)'"); } // // Page Load event handler // protected void Page_Load(object sender, EventArgs e) { createChart(WebChartViewer0, "0"); createChart(WebChartViewer1, "1"); createChart(WebChartViewer2, "2"); createChart(WebChartViewer3, "3"); createChart(WebChartViewer4, "4"); createChart(WebChartViewer5, "5"); createChart(WebChartViewer6, "6"); } </script> <html> <body> <chart:WebChartViewer id="WebChartViewer0" runat="server" /> <chart:WebChartViewer id="WebChartViewer1" runat="server" /> <chart:WebChartViewer id="WebChartViewer2" runat="server" /> <chart:WebChartViewer id="WebChartViewer3" runat="server" /> <chart:WebChartViewer id="WebChartViewer4" runat="server" /> <chart:WebChartViewer id="WebChartViewer5" runat="server" /> <chart:WebChartViewer id="WebChartViewer6" runat="server" /> </body> </html> |
Imports System Imports Microsoft.VisualBasic Imports ChartDirector Public Class donutshading Implements DemoModule 'Name of demo module Public Function getName() As String Implements DemoModule.getName Return "2D Donut Shading" End Function 'Number of charts produced in this demo module Public Function getNoOfCharts() As Integer Implements DemoModule.getNoOfCharts Return 7 End Function 'Main code for creating charts Public Sub createChart(viewer As WinChartViewer, img As String) _ Implements DemoModule.createChart ' The data for the pie chart Dim data() As Double = {18, 30, 20, 15} ' The labels for the pie chart Dim labels() As String = {"Labor", "Licenses", "Facilities", "Production"} ' The colors to use for the sectors Dim colors() As Integer = {&H66aaee, &Heebb22, &Hbbbbbb, &H8844ff} ' Create a PieChart object of size 200 x 220 pixels. Use a vertical gradient ' color from blue (0000cc) to deep blue (000044) as background. Use rounded ' corners of 16 pixels radius. Dim c As PieChart = New PieChart(200, 220) c.setBackground(c.linearGradientColor(0, 0, 0, c.getHeight(), &H0000cc, _ &H000044)) c.setRoundedFrame(&Hffffff, 16) ' Set donut center at (100, 120), and outer/inner radii as 80/40 pixels c.setDonutSize(100, 120, 80, 40) ' Set the pie data c.setData(data, labels) ' Set the sector colors c.setColors2(Chart.DataColor, colors) ' Demonstrates various shading modes If img = "0" Then c.addTitle("Default Shading", "bold", 12, &Hffffff) ElseIf img = "1" Then c.addTitle("Local Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.LocalGradientShading) ElseIf img = "2" Then c.addTitle("Global Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.GlobalGradientShading) ElseIf img = "3" Then c.addTitle("Concave Shading", "bold", 12, &Hffffff) c.setSectorStyle(Chart.ConcaveShading) ElseIf img = "4" Then c.addTitle("Rounded Edge", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RoundedEdgeShading) ElseIf img = "5" Then c.addTitle("Radial Gradient", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RadialShading) ElseIf img = "6" Then c.addTitle("Ring Shading", "bold", 12, &Hffffff) c.setSectorStyle(Chart.RingShading) End If ' Disable the sector labels by setting the color to Transparent c.setLabelStyle("", 8, Chart.Transparent) ' Output the chart viewer.Chart = c 'include tool tip for the chart viewer.ImageMap = c.getHTMLImageMap("clickable", "", _ "title='{label}: US${value}K ({percent}%)'") End Sub End Class |
using System; using ChartDirector; namespace CSharpChartExplorer { public class donutshading : DemoModule { //Name of demo module public string getName() { return "2D Donut Shading"; } //Number of charts produced in this demo module public int getNoOfCharts() { return 7; } //Main code for creating charts public void createChart(WinChartViewer viewer, string img) { // The data for the pie chart double[] data = {18, 30, 20, 15}; // The labels for the pie chart string[] labels = {"Labor", "Licenses", "Facilities", "Production"}; // The colors to use for the sectors int[] colors = {0x66aaee, 0xeebb22, 0xbbbbbb, 0x8844ff}; // Create a PieChart object of size 200 x 220 pixels. Use a vertical // gradient color from blue (0000cc) to deep blue (000044) as background. // Use rounded corners of 16 pixels radius. PieChart c = new PieChart(200, 220); c.setBackground(c.linearGradientColor(0, 0, 0, c.getHeight(), 0x0000cc, 0x000044)); c.setRoundedFrame(0xffffff, 16); // Set donut center at (100, 120), and outer/inner radii as 80/40 pixels c.setDonutSize(100, 120, 80, 40); // Set the pie data c.setData(data, labels); // Set the sector colors c.setColors2(Chart.DataColor, colors); // Demonstrates various shading modes if (img == "0") { c.addTitle("Default Shading", "bold", 12, 0xffffff); } else if (img == "1") { c.addTitle("Local Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.LocalGradientShading); } else if (img == "2") { c.addTitle("Global Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.GlobalGradientShading); } else if (img == "3") { c.addTitle("Concave Shading", "bold", 12, 0xffffff); c.setSectorStyle(Chart.ConcaveShading); } else if (img == "4") { c.addTitle("Rounded Edge", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RoundedEdgeShading); } else if (img == "5") { c.addTitle("Radial Gradient", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RadialShading); } else if (img == "6") { c.addTitle("Ring Shading", "bold", 12, 0xffffff); c.setSectorStyle(Chart.RingShading); } // Disable the sector labels by setting the color to Transparent c.setLabelStyle("", 8, Chart.Transparent); // Output the chart viewer.Chart = c; //include tool tip for the chart viewer.ImageMap = c.getHTMLImageMap("clickable", "", "title='{label}: US${value}K ({percent}%)'"); } } } |