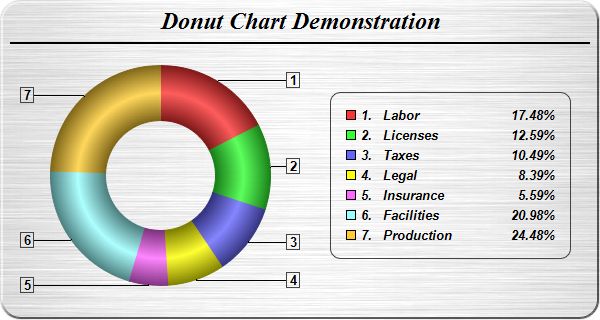
This example demonstrates a donut chart with ring shading effects. It also demonstrates brushed metal background, rounded frame, customized sector labels and using CDML and Parameter Substitution and Formatting to format legend text.
- A donut chart is the same as a pie chart, except that the PieChart.setDonutSize is used instead of PieChart.setPieSize.
- The brushed silver background is achieved by using Chart.brushedSilverColor to create the brushed silver color, then use it in PieChart.PieChart as the chart background color.
- The rounded frame is configured using BaseChart.setRoundedFrame.
- The sector labels are configured using PieChart.setLabelFormat to show the sector number. PieChart.setLabelStyle is used to set the label style. It returns a TextBox object representing the sector label prototype. The Box.setBackground method of the label prototype is then used to add black borders around the labels.
- The legend text is configured by calling the TextBox.setText method of the LegendBox object. The legend text template is specified using CDML and Parameter Substitution and Formatting as follows:
<*block,valign=top*>{={sector}+1}.<*advanceTo=22*><*block,width=120*>{label}<*/*><*block,width=40,halign=right*>{percent}<*/*>%
The starting "<*block,valign=top*>" means the different parts in the legend entry should be aligned to the top. This is necessarily because the second part of the legend entry (described below) can have multiple lines.
The first part of the legend entry is "{={sector}+1}.<*advanceTo=22*>", which means the show the sector number, then "tab" to the 22th pixel position. (In ChartDirector, the sector number starts from 0. So we add 1 to {sector} to make the displayed sector number to start from 1.)
The second part "<*block,width=120*>{label}<*/*>" which means to show the sector label in a 120 pixels block. If the label is wider than 120 pixels, it will be wrapped into multiple lines within the block.
The last part "<*block,width=40,halign=right*>{percent}<*/*>%" means to show the percentage in a 40 pixels block, with the contents right aligned.